Mastering Strategy Patterns: Build Scalable and Flexible Architectures

by
Martin Gutman
on
Oct 7, 2024
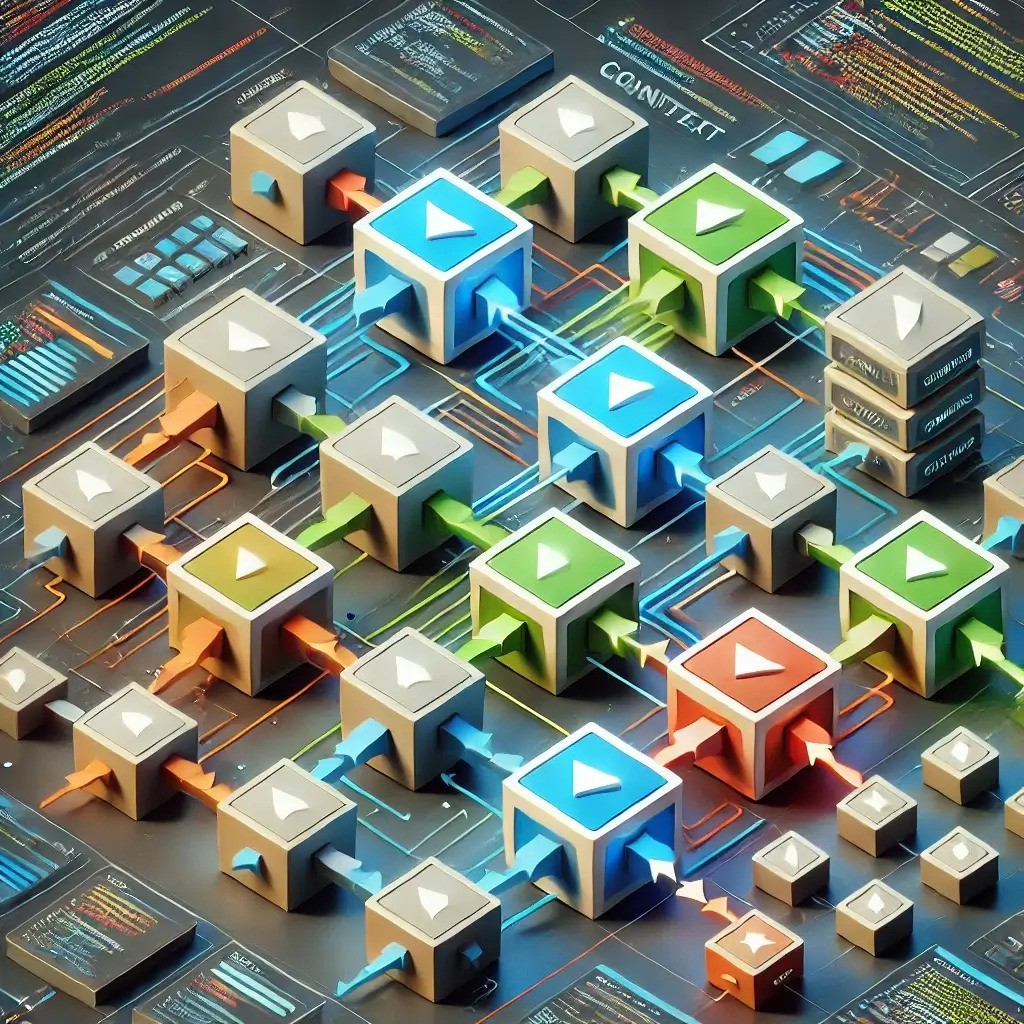
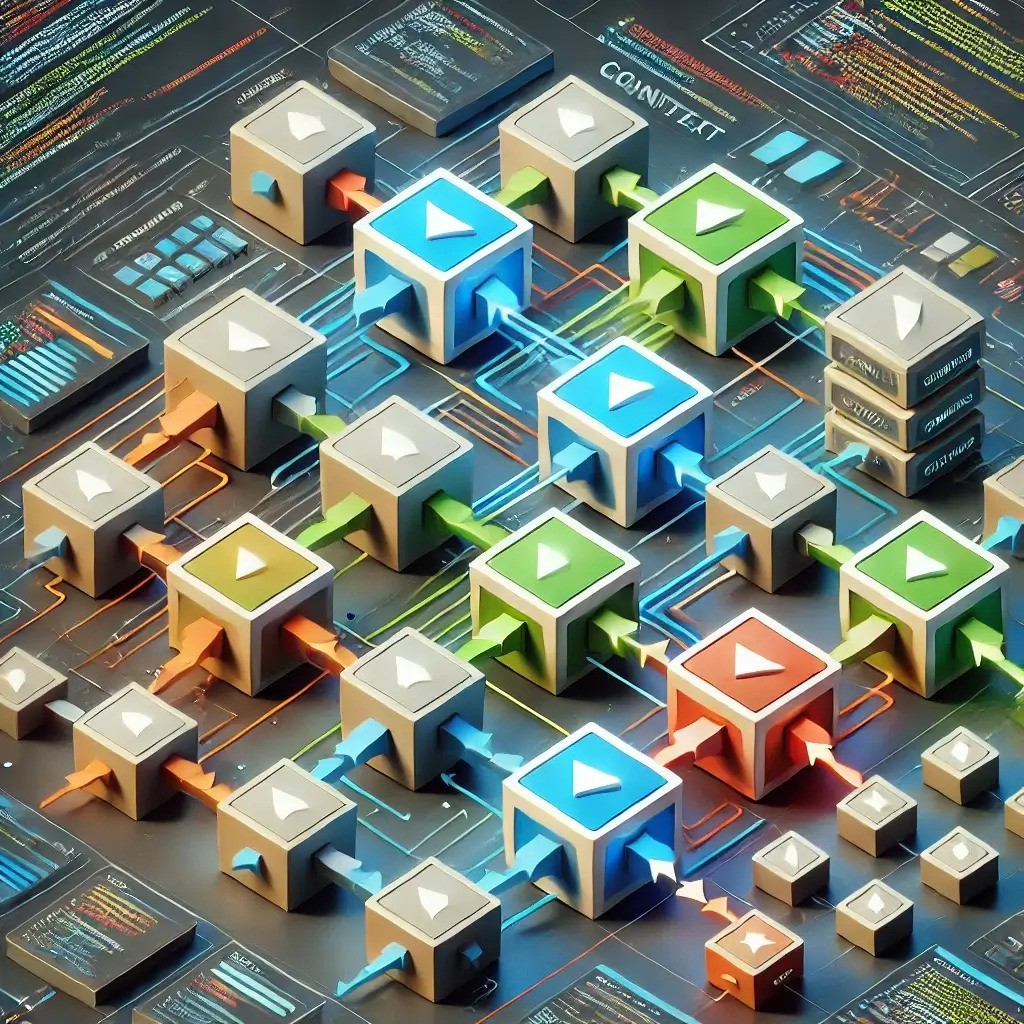
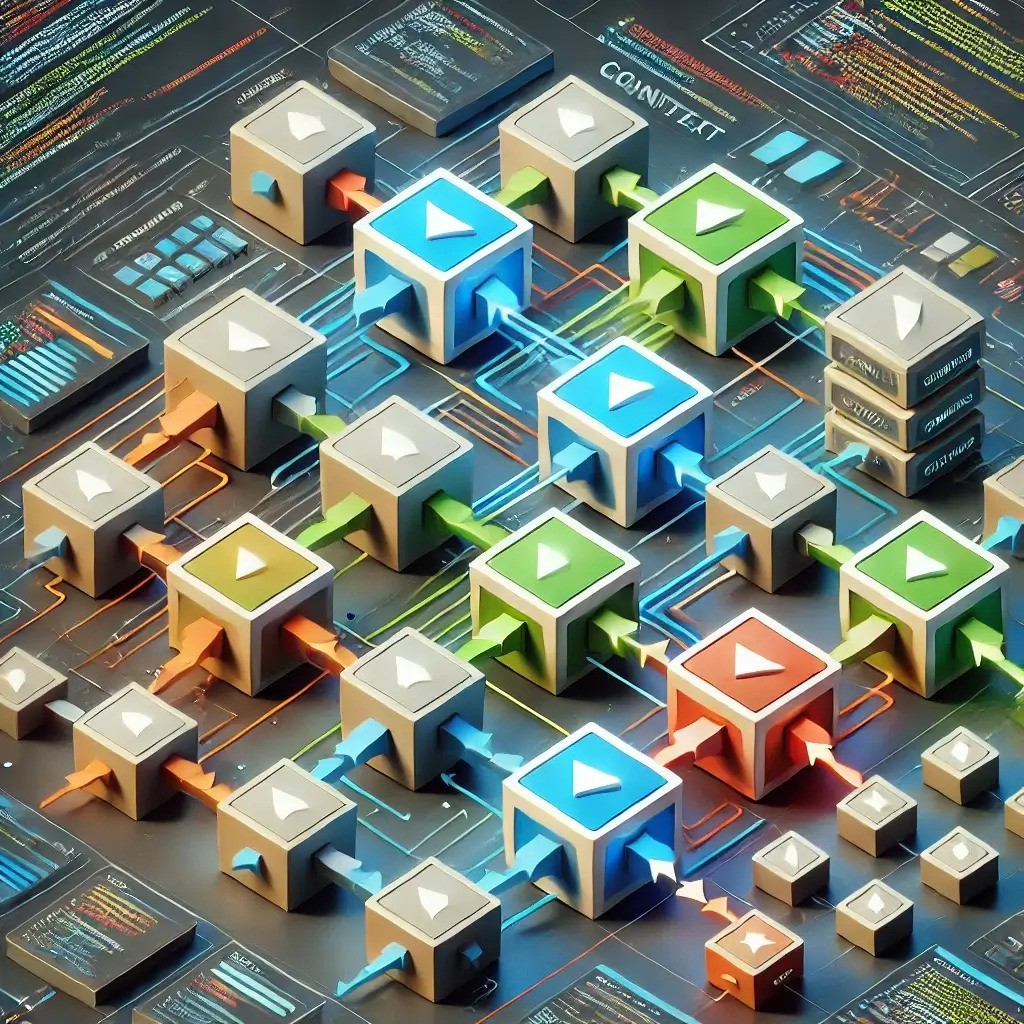
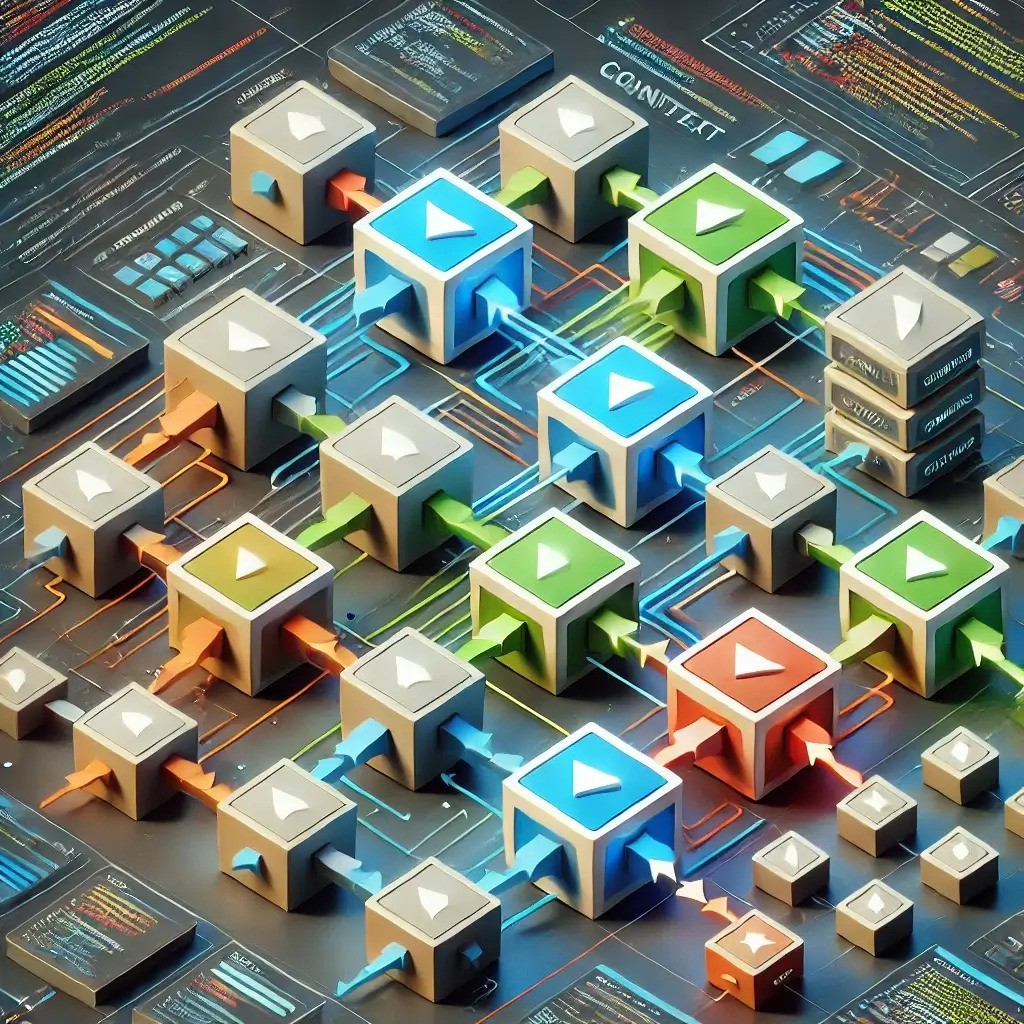
What are Software Design Patterns?
Before we delve into the intricacies of the Strategy Pattern, it’s crucial to understand the concept of software design patterns. In essence, design patterns are tried-and-tested solutions to common problems developers face during software development. They provide a reusable structure that promotes consistency, adaptability, and scalability in a codebase.
Patterns can be categorized into three main types:
Creational patterns: Concerned with object creation mechanisms.
Structural patterns: Deal with class and object composition.
Behavioral patterns: Focus on communication and responsibility between objects.
The Strategy Pattern falls under the behavioral category, where the intent is to define a family of algorithms, encapsulate each one, and make them interchangeable without affecting the overall structure of the application.
Understanding the Strategy Pattern
The Strategy Pattern enables us to define a collection of interchangeable algorithms within a class, allowing the client to choose which algorithm to use at runtime. The key idea here is encapsulation of behavior—meaning that different strategies (algorithms) are stored in separate classes that share a common interface.
In simpler terms, the Strategy Pattern allows you to:
Define a family of algorithms that can be used interchangeably.
Encapsulate each algorithm in its own class.
Separate the algorithm from the code using it, making the codebase more modular.
This pattern shines when you need a scalable way to handle behavior changes without altering the context in which the behavior is executed.
The Key Components of the Strategy Pattern
To fully understand how the Strategy Pattern works, let’s break down its primary components:
Context: The class that uses the strategy. It doesn’t change the way it operates but delegates the task to a strategy object.
Strategy Interface: Defines the contract that all concrete strategies must follow.
Concrete Strategies: These are the implementations of the strategy interface. Each class encapsulates a specific algorithm.
Benefits of Using the Strategy Pattern
When building robust software architectures, the Strategy Pattern offers several key benefits:
Enhanced flexibility: The ability to change strategies at runtime without modifying the context enables dynamic behavior modification. This is especially useful when working on applications where multiple algorithms are required under different conditions.
Better maintainability: Since each strategy is encapsulated in its own class, modifications to one strategy don’t affect others. This reduces the risk of introducing bugs in other areas of the code when changes are made.
Improved testing: Isolating algorithms into their respective classes makes it easier to write unit tests for each strategy.
Separation of concerns: The pattern promotes better organization in the codebase by separating algorithms from the main context. This results in cleaner and more modular code.
Common Use Cases of the Strategy Pattern
The Strategy Pattern can be applied across various real-world scenarios, such as:
1. Payment Processing Systems
Payment systems often need to handle different payment methods like credit cards, PayPal, and cryptocurrency. By employing the Strategy Pattern, each payment method can be encapsulated in its own class, and the payment processor can switch between them dynamically.
2. Sorting Algorithms
Sorting algorithms like quicksort, mergesort, and bubble sort can be encapsulated as strategies. Depending on the dataset, the system can dynamically select the most efficient sorting algorithm without modifying the core sorting logic.
3. Validation Engines
In applications where multiple validation checks are required, each validation rule can be treated as a separate strategy. The validation engine can then execute different rules depending on the input type or context.
4. Logging Frameworks
When building a logging system, you may need to log to different targets (files, databases, or external systems). Each logging mechanism can be treated as a strategy, making it easy to swap between logging targets at runtime.
How to Implement Strategy Patterns in Large-Scale Applications
While the Strategy Pattern can be simple to implement in smaller applications, it’s essential to know how to scale it effectively in large systems. Below are some tips for implementing the pattern in large-scale environments:
1. Use Dependency Injection
In large applications, manually creating and switching between strategies can become cumbersome. By using dependency injection, you can inject the appropriate strategy at runtime, making your system more maintainable and easier to test.
2. Combine with Factory Pattern
To avoid tightly coupling your context to specific strategy implementations, consider combining the Strategy Pattern with the Factory Pattern. This approach allows you to encapsulate the creation logic of different strategies, making your system more scalable and less prone to errors.
3. Design for Extensibility
When designing a system that uses the Strategy Pattern, ensure that new strategies can be easily added without altering the existing ones. This ensures that the system remains flexible and adaptive to future changes.
4. Avoid Overuse
While the Strategy Pattern is incredibly powerful, it’s essential to avoid overusing it. In some cases, simple conditional statements may suffice, and adding too many strategies can lead to unnecessary complexity.
Conclusion
The Strategy Pattern is an invaluable tool for building robust and scalable architectures. It offers flexibility, maintainability, and separation of concerns—core principles for constructing high-quality software systems. By mastering this pattern and implementing it correctly, developers can greatly enhance their application's design and long-term sustainability.
What are Software Design Patterns?
Before we delve into the intricacies of the Strategy Pattern, it’s crucial to understand the concept of software design patterns. In essence, design patterns are tried-and-tested solutions to common problems developers face during software development. They provide a reusable structure that promotes consistency, adaptability, and scalability in a codebase.
Patterns can be categorized into three main types:
Creational patterns: Concerned with object creation mechanisms.
Structural patterns: Deal with class and object composition.
Behavioral patterns: Focus on communication and responsibility between objects.
The Strategy Pattern falls under the behavioral category, where the intent is to define a family of algorithms, encapsulate each one, and make them interchangeable without affecting the overall structure of the application.
Understanding the Strategy Pattern
The Strategy Pattern enables us to define a collection of interchangeable algorithms within a class, allowing the client to choose which algorithm to use at runtime. The key idea here is encapsulation of behavior—meaning that different strategies (algorithms) are stored in separate classes that share a common interface.
In simpler terms, the Strategy Pattern allows you to:
Define a family of algorithms that can be used interchangeably.
Encapsulate each algorithm in its own class.
Separate the algorithm from the code using it, making the codebase more modular.
This pattern shines when you need a scalable way to handle behavior changes without altering the context in which the behavior is executed.
The Key Components of the Strategy Pattern
To fully understand how the Strategy Pattern works, let’s break down its primary components:
Context: The class that uses the strategy. It doesn’t change the way it operates but delegates the task to a strategy object.
Strategy Interface: Defines the contract that all concrete strategies must follow.
Concrete Strategies: These are the implementations of the strategy interface. Each class encapsulates a specific algorithm.
Benefits of Using the Strategy Pattern
When building robust software architectures, the Strategy Pattern offers several key benefits:
Enhanced flexibility: The ability to change strategies at runtime without modifying the context enables dynamic behavior modification. This is especially useful when working on applications where multiple algorithms are required under different conditions.
Better maintainability: Since each strategy is encapsulated in its own class, modifications to one strategy don’t affect others. This reduces the risk of introducing bugs in other areas of the code when changes are made.
Improved testing: Isolating algorithms into their respective classes makes it easier to write unit tests for each strategy.
Separation of concerns: The pattern promotes better organization in the codebase by separating algorithms from the main context. This results in cleaner and more modular code.
Common Use Cases of the Strategy Pattern
The Strategy Pattern can be applied across various real-world scenarios, such as:
1. Payment Processing Systems
Payment systems often need to handle different payment methods like credit cards, PayPal, and cryptocurrency. By employing the Strategy Pattern, each payment method can be encapsulated in its own class, and the payment processor can switch between them dynamically.
2. Sorting Algorithms
Sorting algorithms like quicksort, mergesort, and bubble sort can be encapsulated as strategies. Depending on the dataset, the system can dynamically select the most efficient sorting algorithm without modifying the core sorting logic.
3. Validation Engines
In applications where multiple validation checks are required, each validation rule can be treated as a separate strategy. The validation engine can then execute different rules depending on the input type or context.
4. Logging Frameworks
When building a logging system, you may need to log to different targets (files, databases, or external systems). Each logging mechanism can be treated as a strategy, making it easy to swap between logging targets at runtime.
How to Implement Strategy Patterns in Large-Scale Applications
While the Strategy Pattern can be simple to implement in smaller applications, it’s essential to know how to scale it effectively in large systems. Below are some tips for implementing the pattern in large-scale environments:
1. Use Dependency Injection
In large applications, manually creating and switching between strategies can become cumbersome. By using dependency injection, you can inject the appropriate strategy at runtime, making your system more maintainable and easier to test.
2. Combine with Factory Pattern
To avoid tightly coupling your context to specific strategy implementations, consider combining the Strategy Pattern with the Factory Pattern. This approach allows you to encapsulate the creation logic of different strategies, making your system more scalable and less prone to errors.
3. Design for Extensibility
When designing a system that uses the Strategy Pattern, ensure that new strategies can be easily added without altering the existing ones. This ensures that the system remains flexible and adaptive to future changes.
4. Avoid Overuse
While the Strategy Pattern is incredibly powerful, it’s essential to avoid overusing it. In some cases, simple conditional statements may suffice, and adding too many strategies can lead to unnecessary complexity.
Conclusion
The Strategy Pattern is an invaluable tool for building robust and scalable architectures. It offers flexibility, maintainability, and separation of concerns—core principles for constructing high-quality software systems. By mastering this pattern and implementing it correctly, developers can greatly enhance their application's design and long-term sustainability.
What are Software Design Patterns?
Before we delve into the intricacies of the Strategy Pattern, it’s crucial to understand the concept of software design patterns. In essence, design patterns are tried-and-tested solutions to common problems developers face during software development. They provide a reusable structure that promotes consistency, adaptability, and scalability in a codebase.
Patterns can be categorized into three main types:
Creational patterns: Concerned with object creation mechanisms.
Structural patterns: Deal with class and object composition.
Behavioral patterns: Focus on communication and responsibility between objects.
The Strategy Pattern falls under the behavioral category, where the intent is to define a family of algorithms, encapsulate each one, and make them interchangeable without affecting the overall structure of the application.
Understanding the Strategy Pattern
The Strategy Pattern enables us to define a collection of interchangeable algorithms within a class, allowing the client to choose which algorithm to use at runtime. The key idea here is encapsulation of behavior—meaning that different strategies (algorithms) are stored in separate classes that share a common interface.
In simpler terms, the Strategy Pattern allows you to:
Define a family of algorithms that can be used interchangeably.
Encapsulate each algorithm in its own class.
Separate the algorithm from the code using it, making the codebase more modular.
This pattern shines when you need a scalable way to handle behavior changes without altering the context in which the behavior is executed.
The Key Components of the Strategy Pattern
To fully understand how the Strategy Pattern works, let’s break down its primary components:
Context: The class that uses the strategy. It doesn’t change the way it operates but delegates the task to a strategy object.
Strategy Interface: Defines the contract that all concrete strategies must follow.
Concrete Strategies: These are the implementations of the strategy interface. Each class encapsulates a specific algorithm.
Benefits of Using the Strategy Pattern
When building robust software architectures, the Strategy Pattern offers several key benefits:
Enhanced flexibility: The ability to change strategies at runtime without modifying the context enables dynamic behavior modification. This is especially useful when working on applications where multiple algorithms are required under different conditions.
Better maintainability: Since each strategy is encapsulated in its own class, modifications to one strategy don’t affect others. This reduces the risk of introducing bugs in other areas of the code when changes are made.
Improved testing: Isolating algorithms into their respective classes makes it easier to write unit tests for each strategy.
Separation of concerns: The pattern promotes better organization in the codebase by separating algorithms from the main context. This results in cleaner and more modular code.
Common Use Cases of the Strategy Pattern
The Strategy Pattern can be applied across various real-world scenarios, such as:
1. Payment Processing Systems
Payment systems often need to handle different payment methods like credit cards, PayPal, and cryptocurrency. By employing the Strategy Pattern, each payment method can be encapsulated in its own class, and the payment processor can switch between them dynamically.
2. Sorting Algorithms
Sorting algorithms like quicksort, mergesort, and bubble sort can be encapsulated as strategies. Depending on the dataset, the system can dynamically select the most efficient sorting algorithm without modifying the core sorting logic.
3. Validation Engines
In applications where multiple validation checks are required, each validation rule can be treated as a separate strategy. The validation engine can then execute different rules depending on the input type or context.
4. Logging Frameworks
When building a logging system, you may need to log to different targets (files, databases, or external systems). Each logging mechanism can be treated as a strategy, making it easy to swap between logging targets at runtime.
How to Implement Strategy Patterns in Large-Scale Applications
While the Strategy Pattern can be simple to implement in smaller applications, it’s essential to know how to scale it effectively in large systems. Below are some tips for implementing the pattern in large-scale environments:
1. Use Dependency Injection
In large applications, manually creating and switching between strategies can become cumbersome. By using dependency injection, you can inject the appropriate strategy at runtime, making your system more maintainable and easier to test.
2. Combine with Factory Pattern
To avoid tightly coupling your context to specific strategy implementations, consider combining the Strategy Pattern with the Factory Pattern. This approach allows you to encapsulate the creation logic of different strategies, making your system more scalable and less prone to errors.
3. Design for Extensibility
When designing a system that uses the Strategy Pattern, ensure that new strategies can be easily added without altering the existing ones. This ensures that the system remains flexible and adaptive to future changes.
4. Avoid Overuse
While the Strategy Pattern is incredibly powerful, it’s essential to avoid overusing it. In some cases, simple conditional statements may suffice, and adding too many strategies can lead to unnecessary complexity.
Conclusion
The Strategy Pattern is an invaluable tool for building robust and scalable architectures. It offers flexibility, maintainability, and separation of concerns—core principles for constructing high-quality software systems. By mastering this pattern and implementing it correctly, developers can greatly enhance their application's design and long-term sustainability.
What are Software Design Patterns?
Before we delve into the intricacies of the Strategy Pattern, it’s crucial to understand the concept of software design patterns. In essence, design patterns are tried-and-tested solutions to common problems developers face during software development. They provide a reusable structure that promotes consistency, adaptability, and scalability in a codebase.
Patterns can be categorized into three main types:
Creational patterns: Concerned with object creation mechanisms.
Structural patterns: Deal with class and object composition.
Behavioral patterns: Focus on communication and responsibility between objects.
The Strategy Pattern falls under the behavioral category, where the intent is to define a family of algorithms, encapsulate each one, and make them interchangeable without affecting the overall structure of the application.
Understanding the Strategy Pattern
The Strategy Pattern enables us to define a collection of interchangeable algorithms within a class, allowing the client to choose which algorithm to use at runtime. The key idea here is encapsulation of behavior—meaning that different strategies (algorithms) are stored in separate classes that share a common interface.
In simpler terms, the Strategy Pattern allows you to:
Define a family of algorithms that can be used interchangeably.
Encapsulate each algorithm in its own class.
Separate the algorithm from the code using it, making the codebase more modular.
This pattern shines when you need a scalable way to handle behavior changes without altering the context in which the behavior is executed.
The Key Components of the Strategy Pattern
To fully understand how the Strategy Pattern works, let’s break down its primary components:
Context: The class that uses the strategy. It doesn’t change the way it operates but delegates the task to a strategy object.
Strategy Interface: Defines the contract that all concrete strategies must follow.
Concrete Strategies: These are the implementations of the strategy interface. Each class encapsulates a specific algorithm.
Benefits of Using the Strategy Pattern
When building robust software architectures, the Strategy Pattern offers several key benefits:
Enhanced flexibility: The ability to change strategies at runtime without modifying the context enables dynamic behavior modification. This is especially useful when working on applications where multiple algorithms are required under different conditions.
Better maintainability: Since each strategy is encapsulated in its own class, modifications to one strategy don’t affect others. This reduces the risk of introducing bugs in other areas of the code when changes are made.
Improved testing: Isolating algorithms into their respective classes makes it easier to write unit tests for each strategy.
Separation of concerns: The pattern promotes better organization in the codebase by separating algorithms from the main context. This results in cleaner and more modular code.
Common Use Cases of the Strategy Pattern
The Strategy Pattern can be applied across various real-world scenarios, such as:
1. Payment Processing Systems
Payment systems often need to handle different payment methods like credit cards, PayPal, and cryptocurrency. By employing the Strategy Pattern, each payment method can be encapsulated in its own class, and the payment processor can switch between them dynamically.
2. Sorting Algorithms
Sorting algorithms like quicksort, mergesort, and bubble sort can be encapsulated as strategies. Depending on the dataset, the system can dynamically select the most efficient sorting algorithm without modifying the core sorting logic.
3. Validation Engines
In applications where multiple validation checks are required, each validation rule can be treated as a separate strategy. The validation engine can then execute different rules depending on the input type or context.
4. Logging Frameworks
When building a logging system, you may need to log to different targets (files, databases, or external systems). Each logging mechanism can be treated as a strategy, making it easy to swap between logging targets at runtime.
How to Implement Strategy Patterns in Large-Scale Applications
While the Strategy Pattern can be simple to implement in smaller applications, it’s essential to know how to scale it effectively in large systems. Below are some tips for implementing the pattern in large-scale environments:
1. Use Dependency Injection
In large applications, manually creating and switching between strategies can become cumbersome. By using dependency injection, you can inject the appropriate strategy at runtime, making your system more maintainable and easier to test.
2. Combine with Factory Pattern
To avoid tightly coupling your context to specific strategy implementations, consider combining the Strategy Pattern with the Factory Pattern. This approach allows you to encapsulate the creation logic of different strategies, making your system more scalable and less prone to errors.
3. Design for Extensibility
When designing a system that uses the Strategy Pattern, ensure that new strategies can be easily added without altering the existing ones. This ensures that the system remains flexible and adaptive to future changes.
4. Avoid Overuse
While the Strategy Pattern is incredibly powerful, it’s essential to avoid overusing it. In some cases, simple conditional statements may suffice, and adding too many strategies can lead to unnecessary complexity.
Conclusion
The Strategy Pattern is an invaluable tool for building robust and scalable architectures. It offers flexibility, maintainability, and separation of concerns—core principles for constructing high-quality software systems. By mastering this pattern and implementing it correctly, developers can greatly enhance their application's design and long-term sustainability.
Your LatAm dev partner
Join fast-moving companies
that hire Build Online developers
to build more for less.
© Copyright 2024, All Rights Reserved
Join fast-moving companies
that hire Build Online developers
to build more for less.
© Copyright 2024, All Rights Reserved
Join fast-moving companies
that hire Build Online developers
to build more for less.
© Copyright 2024, All Rights Reserved
Join fast-moving companies
that hire Build Online developers
to build more for less.
© Copyright 2024, All Rights Reserved